Table of Contents
Last update on
If you use the WordPress REST API, securing your endpoints is a crucial step to keep them from becoming attack vectors. In this guide, we are exploring best practices to do so.
Below, we are discussing how to keep API traffic safe, set the right permissions, protect and clean transmitted data, and stay on top of potential issues.
TL;DR for WordPress API Endpoints
Pressed for time? Here are the main takeaways.
The WordPress REST API allows powerful integrations and flexibility, but its endpoints can also open doors to attacks if left unsecured. To keep your site safe, it’s important to implement layered security for every aspect of API usage:
- Keep WordPress, its themes, and plugins up to date to patch known vulnerabilities.
- Secure all API traffic with SSL/HTTPS to protect against interception and tampering.
- Prioritize site speed to avoid timeouts, process API calls quickly, and keep your site working during denial-of service attacks.
- Use custom user roles and permissions to keep privileges to the essential minimum.
- Validate and sanitize data to keep out malicious markup.
- Use authentication and authorization to control API endpoint access.
- Add rate limiting to defend against brute-force, scraping, and DoS attacks.
- Harden your setup with security headers, logging, and IP whitelisting where needed.
Getting to Know the WordPress REST API
Before diving into security practices for custom API endpoints, it’s important to understand what they are and how they fit into the WordPress ecosystem.
What Is the WordPress REST API?
Let’s start at the very top. API stands for “Application Programming Interface.” It’s a piece of software that allows two different programs to communicate with each other and exchange data.
WordPress has many APIs, but the most powerful and important is the REST API. It’s been a core feature since WordPress 4.7.
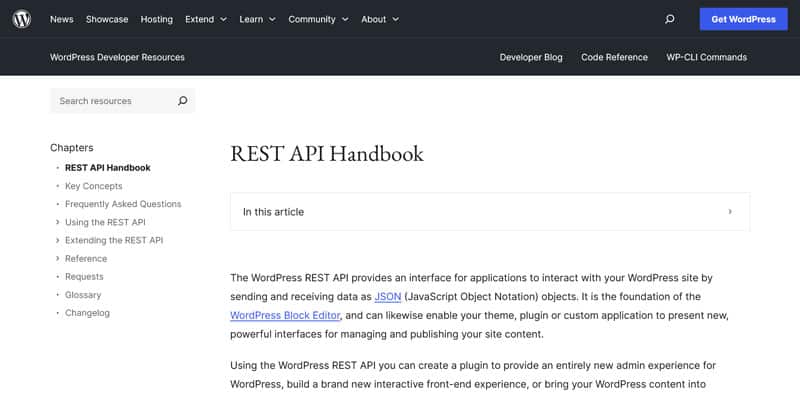
REST stands for “Representational Sate Transfer” and describes a standard for creating APIs that offers several benefits. One of them is that RESTful APIs have an easier time communicating with each other.
The WordPress REST API also uses the JSON (JavaScript Object Notation) data format, which is easy to read and write and compatible with many programming languages.
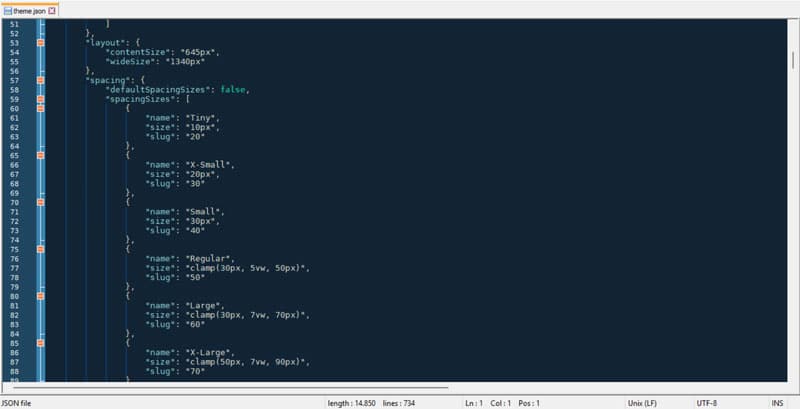
It provides a standardized way to interact with WordPress using HTTP requests like GET (retrieve), POST (create), PUT/PATCH (update), and DELETE (remove). With their help, it’s possible to manipulate core data types like posts, pages, users, and comments as well as custom content types, from outside WordPress without logging into the website first.
All of this makes it possible to integrate and connect WordPress with a wide range of external software, tools, and applications. For example, the REST API is a foundational tool for headless WordPress setups or integrating your website with mobile apps. The WordPress block editor (aka Gutenberg) also works using the REST API.
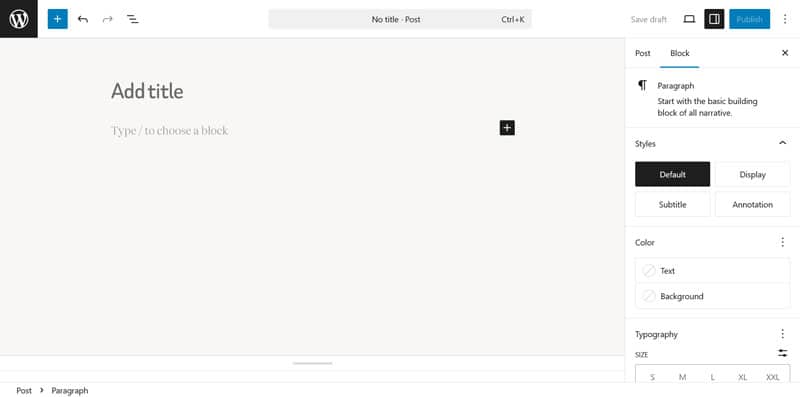
What Are API Endpoints in WordPress?
An endpoint is a specific URL that represents an object or a resource in your WordPress site. It could be a post, a user, or a custom setting. When another piece of software sends a request to that URL, it may cause WordPress to provide or modify the data located at that endpoint.
WordPress has built-in endpoints for default data types like posts, comments, media, and users. You can also create custom endpoints for tailored functionality, to access custom data, limit data exposure, or streamline performance. This is useful, for example to:
- Allow external apps to make changes like publishing or retrieving posts and updating user profiles.
- Connect WordPress to third-party tools, apps, and services (e.g., CRMs, email marketing providers).
- Enable real-time data fetching for dynamic website features, such as live search.
- Build custom admin panels or analytics dashboards.
Why Is API Endpoint Security Important?
Because the API offers direct access to website data, it also brings security risks with it. Every API endpoint is a potential entry point into your WordPress site. Without proper security measures, they can be exploited and cause damage, such as:
- Reveal sensitive information
- Enable brute force or injection attacks
- Expose admin functions
- Open corridors for denial-of-service (DoS) attacks
- Make malware injection possible
For example, when you access https://yoursite.com/wp-json/wp/v2/users/ (replace yoursite.com with your real domain), by default, you will see a list of all the users available on your website.
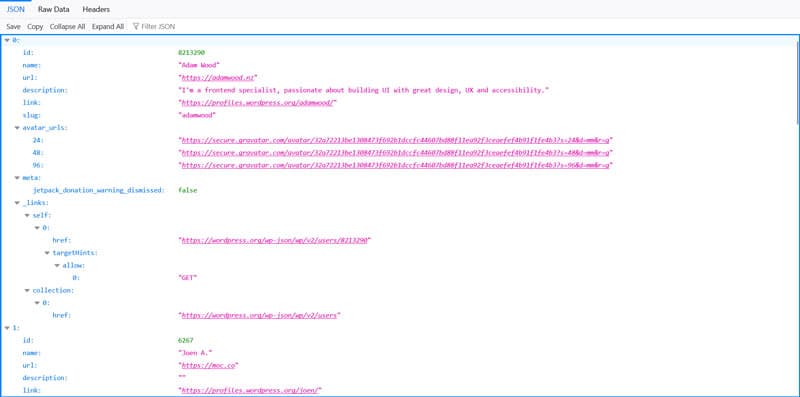
This makes it easier for hackers to brute force their way into your site, because it provides them half of the credentials needed to log in. That’s why it’s important to know how to secure your API endpoints to keep your WordPress website safe.
But doing so isn’t about a single fix. It requires a combination of strategies, which we’ll talk about now.
1. Apply Software Updates Regularly
Keeping WordPress core, plugins, and themes updated applies the latest patches for known security vulnerabilities. You can apply them manually or use the automatic update function in WordPress.

By default, minor core updates already install automatically as they are often released specifically to address security issues. When you apply major updates, be sure to back up your site first and test them out on a staging website.
In addition, don’t forget to review and update any custom plugins or code that handle API routes.
2. Use SSL/HTTPS
Implementing SSL on your website ensures that all API requests and responses are encrypted in transit. This protects sensitive data like authentication tokens or user information from being intercepted or manipulated by man-in-the-middle attacks.
To run your website on HTTPS, you need an SSL/TLS certificate. If your website doesn’t have that yet, it’s usually easiest to set up through your hosting provider. After that, you still need to enforce HTTPS usage for all traffic and API calls.
3. Invest in Website Performance
Just like all other parts of your website, for the REST API to do its job, it needs good site performance. If your website can’t accommodate API requests because of a lack of server resources, the applications and integrations that depend on them can experience slow responses and timeouts.
In addition, a speed-optimized website has a better chance to stay responsive for visitors and fixes during an attack on API endpoints aiming to overload it.
There are many ways to improve website performance, such as investing in quality hosting and optimizing your images. An easy way to speed up your WordPress site is to use a performance plugin like WP Rocket. It includes many features to increase site speed like:
- Caching, including a separate mobile cache
- Lazy loading for images, including CSS backgrounds, videos, and iframes
- Deferring render-blocking resources
- Preloading cache, links, external files, and fonts
- Database optimization
Aside from that, WP Rocket implements additional performance improvements in the background, like GZIP compression, minifying CSS and JavaScript files, and image optimization above the fold (to improve Largest Contentful Paint).
As a result, your site fulfills 80% of performance best practices as soon as you activate the plugin and gets faster immediately without any extra work on your end.
4. Set up Custom User Roles
WordPress has user roles with defined capabilities as a default feature.
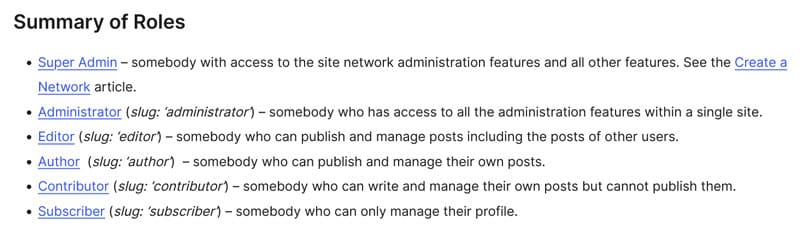
Because API requests use authentication credentials belonging to users on your website, setting the right access level also determines what endpoints they can use and what operations they can perform:
- Administrator: Can perform all CRUD (Create, Read, Update, Delete) operations on all API endpoints.
- Editor: Has access to most API endpoints related to posts, pages, and media, with permissions to create, edit, and delete content.
- Author: Includes access to endpoints for creating and updating posts assigned to the user.
- Contributor: Access to API endpoints is limited to their own drafts without the ability to publish them.
- Subscriber: Subscribers are typically only able to retrieve their own user profile information.
For API endpoint security, avoid using high-privilege roles (e.g., administrator) if not necessary for the task at hand. This reduces the possible fallout from a potential breach.
Better yet, create dedicated roles with only the permissions needed for what you are trying to achieve. This is also called the principle of least privilege.
You can use the add_role() and add_cap() functions to define custom roles and manage capabilities as well as remove_cap() to take away privileges from existing user roles. Alternatively, use the User Role Editor plugin.
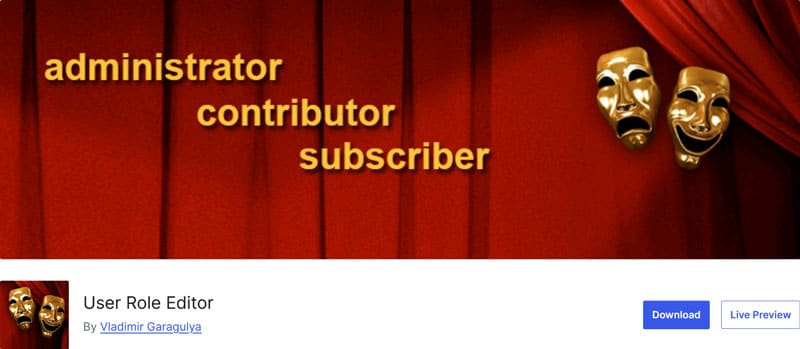
It’s also possible to completely disable the REST API for non-authorized users using a plugin like Disable WP REST API or via functions.php.
5. Implement Input Validation and Sanitization
Input validation and data sanitization keep attackers from submitting harmful SQL commands or scripts via API endpoints.
Validation means checking incoming data to ensure it matches expected formats, types, and allowed values. Sanitization, on the other hand, removes harmful code embedded in requests. Both are especially important for user-generated content.
WordPress offers several native functions for this purpose that you can find in the developer resources:
6. Utilize Prepared Statements for Database Queries
Prepared statements keep user input and database commands separate. That way, they help prevent SQL injection attacks by neutralizing malicious query structures.
In WordPress, you can use $wpdb->prepare() when building SQL queries to automatically escape user input as well as include placeholders (%s, %d, etc.) instead of directly inserting raw values into queries.
7. Ensure Strong Authentication and Authorization Practices
Authentication makes sure users or entities who request data from an API endpoint are who they claim to be. Authorization, on the other hand, controls what a given user has access to and can do. We already covered this when talking about user roles earlier.
By default, the WordPress REST API offers authentication via cookies and nonces, which are unique, short-lived tokens. You can also use basic authentication by setting an application password in your user profile.
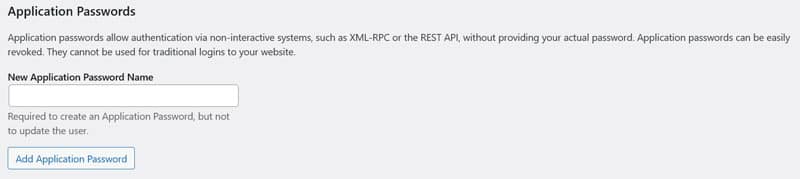
OAuth and JSON Web Tokens are also available through plugins like WP REST API – OAuth 1.0a Server and JWT Authentication for WP REST API.
Use the different types of authentication in the following cases:
- Cookies + Nonces: Ideal for themes/plugins making API calls in the browser.
- Application Passwords (Basic Auth): Use for external scripts, tools, or services. It’s easy to set up, and good for server-to-server communication.
- OAuth: Best for third-party apps that need user-granted access.
- JWT (JSON Web Tokens): Good for headless or decoupled front ends (e.g., React or mobile apps) and API endpoints with high traffic.
8. Employ Rate Limiting and Throttling
Rate limiting allows you to set limits on how many requests a user or IP can make in a given time period. It helps defend against brute-force attacks, spam, automated abuse aiming to overload the system overload.
Rate limiting is especially important for public or unauthenticated endpoints. At the same time, it’s not so restrictive that it blocks all legitimate requests and traffic when under attack.
Limits can be placed by client or location or be put on the server itself. You can use hard limits, which disallow any requests until lifted, or throttle the number of requests during a time period. Finally, you can also set different limits for different user groups.
WP tutorials has a great guide on rate limiting for the WordPress API.
9. Use Security Headers
Security headers provide an extra layer of protection by instructing browsers how to handle your site’s content and connections. When your API interacts with front-end apps or external services, these headers help mitigate common web vulnerabilities.
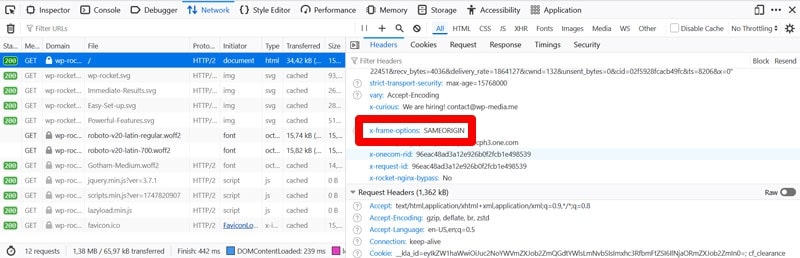
Important security headers to implement are:
- Content-Security-Policy: Controls which scripts can run in API-connected front ends.
- X-Content-Type-Options: Set it to nosniff to prevent browsers from interpreting files as a different MIME type.
- X-Frame-Options: Using DENY or SAMEORIGIN helps you prevent clickjacking by forbidding your site from being embedded in an iframe.
- Strict-Transport-Security: Enforce HTTPS on future requests.
Security headers can be added using PHP via the header() function, through server files like .htaccess, or security plugins such as Headers Security Advanced. Learn more about security headers.
10. Allowlist IP Addresses
Another way to control access to sensitive or admin endpoints is by restricting it to specific, predefined IP addresses or ranges. This acts as a simple but effective barrier against unauthorized requests from unknown sources.
Of course, the prerequisite is that you know the IP addresses of the devices and programs you want to allow to access your API endpoints and that they stay static. You can configure allowlists at the server level (e.g., .htaccess, NGINX config), via functions.php, and some security plugins.
11. Take Advantage of Logging and Monitoring
Finally, recording API requests, responses, and user activity is another great tool to secure WordPress API endpoints. It allows you to identify weak points, monitor request patterns, spot errors, and pinpoint the source of security breaches.
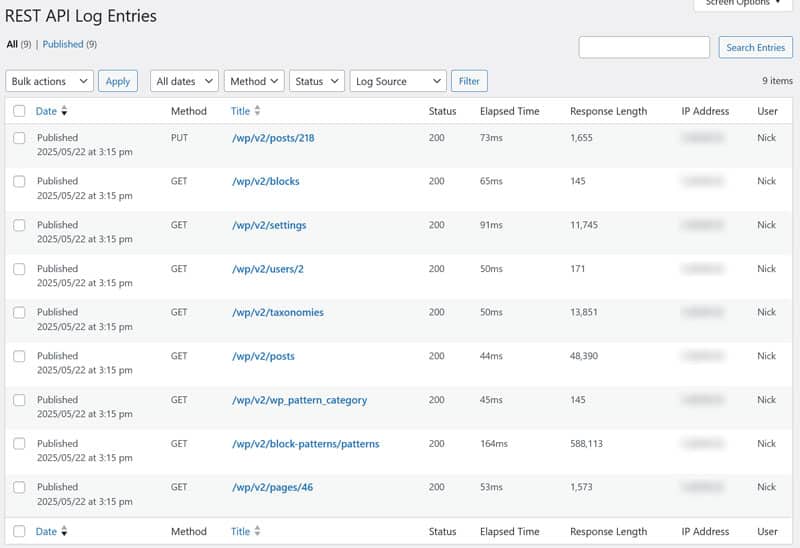
This helps strengthen your security over time. You can use a plugin like REST API Log, your server access logs, or custom logging functions.
Secure Your Custom WordPress API Endpoints Now
The WordPress REST API and its endpoints are powerful but can pose serious risks if not properly secured. Making them safe to use requires different layers and a multi-pronged approach. But, as you have seen above, it’s relatively straightforward.
One aspect you shouldn’t overlook is site performance. A well-optimized site can deliver data via API faster and has a higher chance of staying responsive for troubleshooting during a denial-of-service attack. For an easy and user-friendly way to speed up your site, WP Rocket!