Table of Contents
Last update on
At Elephant, we’re using WP Rocket to cache our WordPress WooCommerce websites. Simple to set up, full of features and good compatibility with most of the commonly used plugins (ACF, WPML, etc) in the WordPress realm.
WP Rocket uses all kinds of different ways to speed up your site, from saving static pages in wp-content/cache/wp-rocket (gzipped for faster download), combining and concatenating JS and CSS (if you want it to), to adding headers to your .htaccess.
This makes the whole loading process of your site very quick, though with webshops, this might be a bit problematic, since you need them to show some new stuff every now and then, right?
WP Rocket allows you to exclude any page(s) from the cache, but when you’re using WooCommerce, Easy Digital Download, iThemes Exchange, Jigoshop or WP-Shop, you’ll be fine since cart and checkout pages will be auto-excluded from those plugins automatically.
Recently, however, I ran into a little problem with the mini cart that WooCommerce offers and that you can use, for example, in your webshop header. The mini cart was not Ajax-ed, so the content was being cached and sometimes made it look like you didn’t have any items in your cart even when you did. By reaching out via Twitter I found out that the only way to solve this correctly was by retrieving this mini cart via WP AJAX. Since I promised the helpful team at WP Rocket a write up, I’ll tell you how I did this.
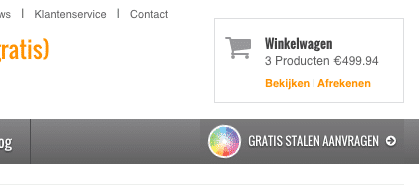
The mini cart over at jaloezieenverkoop.nl has only one dynamic part: {x} product(s) {total_price}. You can generate a mini cart using the Woo function woocommerce_mini_cart(), but for me, this proved to be a bit too much information.
So, my mini cart looks like this:
Please note the span .cart-result – that’s the span we’re going to use for our mini cart data. The rest of our mini cart is always going to be the same (checkout button, go to cart button), so we can easily have those elements cached.
We setup the AJAX call next. I’m using regular jQuery, obviously there are other ways to do this, but I suspect that most of you out there are comfortable with this way of doing things.
So first, the JS initializing the call:
As you can see we’re checking to see if .cart-result is there, we create the $_POST data (our only data being the call we want to make) and we re-use the WooCommerce AJAX URL so we don’t have to enqueue it ourselved.
With the data returned, we fill up .cart-result. Nothing more, nothing less.
Next step, let’s see what our PHP looks like…
We add some actions here that receive the calls made by our Javascript. I’m not diving in on how that works (you could check that yourselves in the WordPress codex, but it basically only returns the template part with the cart data we need: {x} product(s) {total_price}. I’m working with template parts here and I think you should to – it’s best practice not having your markup tangled up with your code, of course (if I did, I’d have some evil front end devs harassing me in no time).
The part looks like this:
I’m using Woo’s global to retrieve the data I need and only that, not the total mini cart you get from woocommerce_mini_cart() – though that might be nice to retrieve here as well, if you need that kind of data.
And that’s it! Your cart will always contain the right data and WP Rocket will only cache the parts it needs to cache. Now, there is one little catch here, if you’re on a slower webserver; and that is the AJAX delay in loading that bit of data. I always find myself using jQuery Block UI to provide a loading message until the data is returned to provide an optimal user experience – but that is a bit out of the scope of this blog.
Good luck!